Reverse list elements in C#
Overview
Background
.NET core provides a generics class List to store a strongly types objects that can be accessed by index.
This class provides us with many methods to add, remove, access, sort or manipulate the objects within the list.
In this sample, I am going to demonstrate the following Reverse
options:
- Reverse using the
System.Collections.Generic List
's methods. - Reverse using
Linq
method
Code Samples
List Initialization
I am going to create a list of integers and set the values using a collection initializer.
1var list = new List<int>
2{
3 1, 5, 6, 7, 9, 10, 99, 777
4};
Note that using a collection initializer as shown above produces the same code as separately using the Add function multiple times:
1var list = new List<int>();
2list.Add(1);
3list.Add(5);
4list.Add(6);
5list.Add(7);
6list.Add(9);
7list.Add(10);
8list.Add(99);
9list.Add(777);
Printing to console the original list, produces the following output:
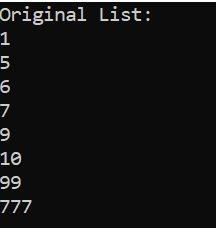
Original list of items sample output
Reverse using List Reverse Methods
The name of the method is self-explanatory – it reverses the order of the elements in the list.
Important note: The Reverse methods are reversing the list in-place, meaning your original List object is being changed.
The Reverse method has 2 overloads:
Reverse(void)
- Reverses the all the elements in the given listReverse(int, int)
- Reverses the order of the elements in the specified range
Full reverse in-place
1list.Reverse();
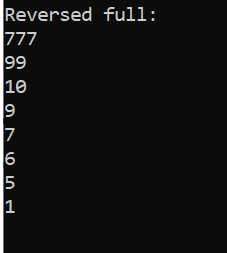
Reverse full list in C#
Partial reverse in-place
1list.Reverse(0, 3)
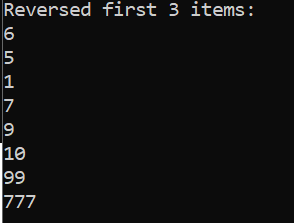
Reverse first 3 items in C# List
Reverse using Linq Reverse Method
In case your wish to keep the original list unchanged, the following Linq code will create another list with reversed items:
1list.AsEnumerable().Reverse();
This is also available as query syntax:
1(from i in list select i).Reverse();
Full code:
1public class Program
2{
3 static void Main(string[] args)
4 {
5 // initialize list.
6 var list = new List<int>
7 {
8 1, 5, 6, 7, 9, 10, 99, 777
9 };
10
11 PrintList("Original List:", list);
12
13 list.Reverse();
14 PrintList("Reversed full:", list);
15 list.Reverse(); // reverse back since the list is changed.
16
17 // reverse first 3 items.
18 list.Reverse(0, 3);
19 PrintList("Reversed first 3 items:", list);
20 list.Reverse(0, 3); // reverse back.
21
22
23 PrintList("Reversed Using LINQ full:", list.AsEnumerable().Reverse());
24
25 PrintList("Reversed Using LINQ Query Syntax:", (from i in list select i).Reverse());
26 }
27
28 static void PrintList<T>(string message, IEnumerable<T> list)
29 {
30 Console.WriteLine($"{message}\r\n{string.Join("\r\n", list)}");
31 }
32}