C# - How to add or remove items from Windows recent files
Overview
Background
Starting Windows 7, Microsoft added a capability for displaying recently used files. This usually includes documents, pictures, and movies we've recently accessed. These files can be seen in various Windows components, including:
- Recent files
- Recent items
- Start menu or application's Jump List
The management of the listed files is done by the operating system.
In this post, I will show how to programmatically add and remove items from the Recent files list using C#.
My Stack
- Visual Studio 2019 Community Edition (16.5.1)
- Console application built on .NET Framework 4.7.2 (C#) – 32/64 bit.
- Windows 10 Pro 64-bit (10.0, Build 18363) (18362.19h1_release.190318-1202)
Solution
- I created a helper class that uses Windows Shell API SHAddToRecentDocs.
- Imported the function using PInvoke.
- Added 2 functions:
- AddFile -> adds the file to Recent files view.
- ClearAll -> clears all files from Recent files view.
1public static class RecentDocsHelpers
2{
3 public enum ShellAddToRecentDocsFlags
4 {
5 Pidl = 0x001,
6 Path = 0x002,
7 PathW = 0x003
8 }
9
10 [DllImport("shell32.dll", CharSet = CharSet.Unicode)]
11 private static extern void SHAddToRecentDocs(ShellAddToRecentDocsFlags flag, string path);
12
13 public static void AddFile(string path)
14 {
15 SHAddToRecentDocs(ShellAddToRecentDocsFlags.PathW, path);
16 }
17
18 public static void ClearAll()
19 {
20 SHAddToRecentDocs(ShellAddToRecentDocsFlags.Pidl, null);
21 }
22}
Usage
1class Program
2{
3 static void Main(string[] args)
4 {
5 RecentDocsHelpers.ClearAll();
6
7 // add c:\temp\sample.json to recent files.
8 RecentDocsHelpers.AddFile(@"c:\temp\sample.json");
9 }
10}
Limitation
- You can not add executable files to Recent files.
Result
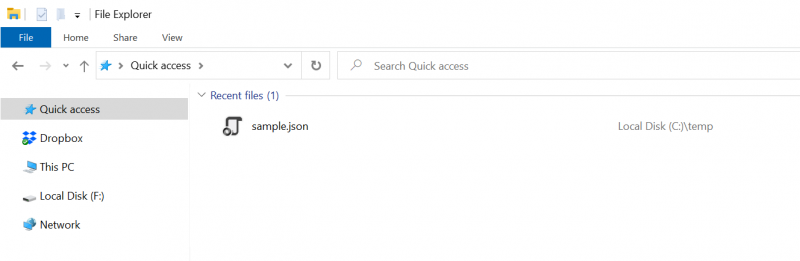
Windows recent files
Useful resources
- Source code of this project on GitHub